Hi, I introduce my very small project that I decide to describe in my blog as first entry.
I come up with idea of image browser when I dowloaded picture from diferent devices like camera, mobile phone etc. into my home library. Each device save name of picture in unlike way e.g.
- IMG_4953
- 20240916_184140
- DSC00769
It is of course frustrating when I want to display images in date order, especially when I try to display on TV set to my familly. Comonlly image browser display images in alphabetical order, not in created date.
Photo Organizer
In this way I decided to create simple script to organize names of photos in Java. Structure of the project should be smoething like this:
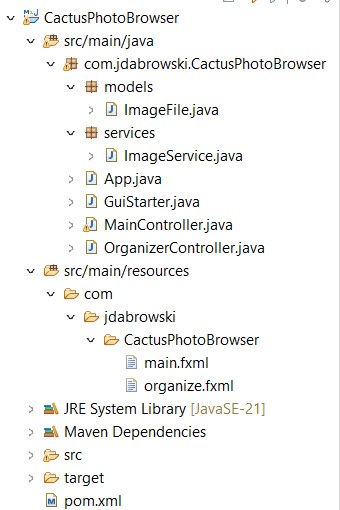
At first I create ImageFile
class for storage data of image file.
public class ImageFile {
private String name;
private FileTime date;
private Path path;
public String getName() {
return name;
}
public ImageFile() {
super();
}
public ImageFile(String name, FileTime date) {
super();
this.name = name;
this.date = date;
}
//Geters and setters
}
I next step I created ImageService
class with two method:
public ArrayList<ImageFile> imagesList(String directory) {
ArrayList<ImageFile> imageFiles = new ArrayList<>();
// Path to file
Path path = Paths.get(directory);
// Load files list
try (DirectoryStream<Path> stream = Files.newDirectoryStream(path, "*.{jpg,JPG}")) {
for (Path entry : stream) {
// Get file created date
BasicFileAttributes attrs = Files.readAttributes(entry, BasicFileAttributes.class);
FileTime creationDate = attrs.lastModifiedTime();
// Add file into list with created date
imageFiles.add(new ImageFile(entry.getFileName().toString(), creationDate));
}
} catch (IOException e) {
e.printStackTrace();
}
return imageFiles;
}
First method is imagesList
, the function returns list of image files object ArrayList<ImageFile>
.
As argument imageList takes path to folder. As you can see I use only .jpg files DirectoryStream<Path> stream = Files.newDirectoryStream(path, "*.{jpg,JPG}")
, I think other extensions like .png etc. isn’t a priory, especially when I want to organize photos.
public void saveFileList(ArrayList<ImageFile> fileList, String directory, String prefix) {
String path = directory+"//OrganizedPhotos";
int i = 1;
new File(path).mkdirs();
for (ImageFile imageFile : fileList) {
Path sourcePath = Paths.get(directory+"//"+imageFile.getName());
Path targetPath = Paths.get(path+"//"+prefix+"_"+i+".JPG");
try {
Files.copy(sourcePath, targetPath);
} catch (IOException e) {
e.printStackTrace();
}
i++;
}
}
Next method is saveFileList
, the function save all files from provide file list. Method don’t affect files, just copy files into new folder called “OrganizedPhotos” with rename using prefix. Basically this is all we need for solve this problem, but at this stage I thought it would be nice to put the photo organizer as tool into photo browser.
In next step I create GUI interface to handle my feature:
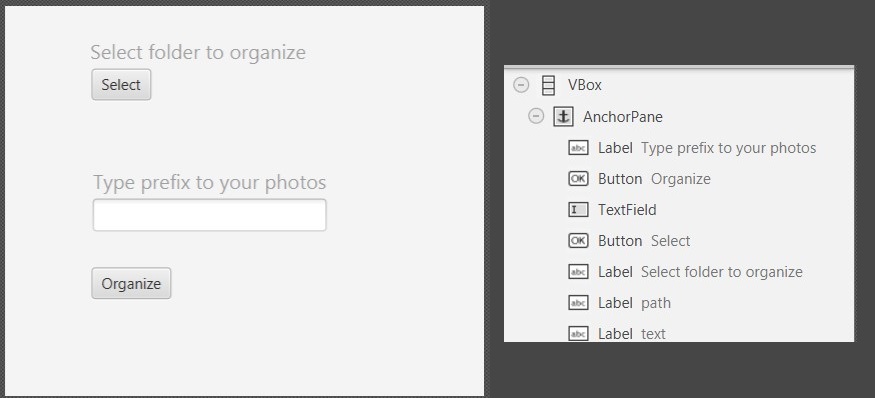